As the topic itself elaborate on what we will be handling in this article now.
During my AEM SPA implementation I encountered one issue where I needed something like column control in SPA architecture. But as you know earlier we used to have HTL rendered component, so it was easy to handle the division and crating a parsys inside parsys.
But as we are using SPA architecture we should have all rendering at React end.
Let’s understand how we can achieve the same thing in current SPA architecture.
Create a basic AEM component under your project structure let’s say /apps/mycompany/content/components/mygrid with resourceSupertype as wcm/foundation/components/responsivegrid. (overlaying)
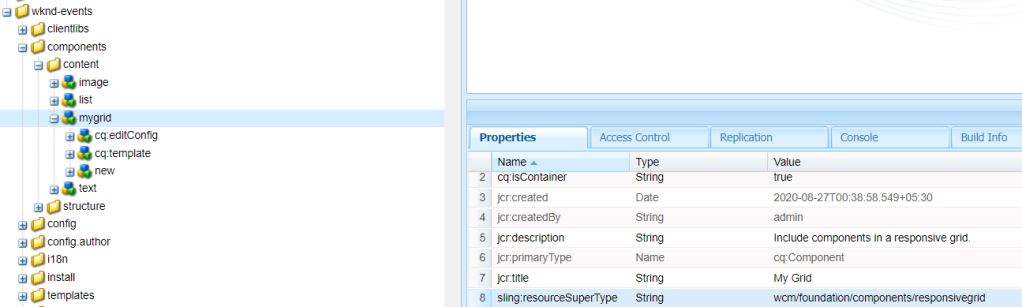
Now we start with creating our corresponding React component inside ../react-app/src/components/MyGrid/Mygrid.js
I have used the following JS and CSS to extend the ResponsiveGrid class from @adobe/cq-react-editable-components.
MyGrid.js
import React, { Component } from "react";
import {ResponsiveGrid, MapTo, withComponentMappingContext} from "@adobe/cq-react-editable-components";
require('./MyGrid.scss');
export class MyGrid extends ResponsiveGrid {
/**
* The attributes that will be injected in the root element of the container
*
* @returns {Object} - the attributes of the container
*/
get containerProps() {
let containerProps = super.containerProps;
containerProps.className = (containerProps.className || '') + ' MyGrid ' + this.props.gridClassNames;
return containerProps;
}
render() {
return (
<div {...this.containerProps}>
{ super.childComponents }
{ super.placeholderComponent }
</div>
)
}
}
MapTo('wknd-events/components/content/mygrid')(withComponentMappingContext(MyGrid));
MyGrid.scss
@import '../../styles/shared';
.MyGrid{
padding: $gutter-padding;
width: 50%;
p {
color: $color-white;
}
&-message {
color: $color-primary;
}
}
Also place the following SCSS files at ../react-app/src/styles
shared.scss
@import './variables';
//Mixins
@mixin media($types...) {
@each $type in $types {
@if $type == tablet {
@media only screen and (min-width: $small-screen + 1) and (max-width: $medium-screen) {
@content;
}
}
@if $type == desktop {
@media only screen and (min-width: $medium-screen + 1) {
@content;
}
}
@if $type == mobile {
@media only screen and (min-width: $mobile-screen + 1) and (max-width: $small-screen) {
@content;
}
}
}
}
@mixin content-area () {
max-width: $max-width;
margin: 0 auto;
padding: $gutter-padding;
}
@mixin component-padding() {
padding: 0 $gutter-padding !important;
}
@mixin drop-shadow () {
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19);
}
variables.scss
//Typography
$em-base: 20px;
$base-font-size: 1rem;
$small-font-size: 1.4rem
;
$lead-font-size: 2rem;
$title-font-size: 5.2rem;
$h1-font-size: 3rem;
$h2-font-size: 2.5rem;
$h3-font-size: 2rem;
$h4-font-size: 1.5rem;
$h5-font-size: 1.3rem;
$h6-font-size: 1rem;
$base-line-height: 1.5;
$heading-line-height: 1.3;
$lead-line-height: 1.7;
$font-serif: ‘Asar’, serif;
$font-sans: ‘Source Sans Pro’, sans-serif;
$font-weight-light: 300;
$font-weight-normal: 400;
$font-weight-semi-bold: 600;
$font-weight-bold: 700;
//Colors
$color-white: #ffffff;
$color-black: #080808;
$color-yellow: #FFEA08;
$color-gray: #808080;
$color-dark-gray: #707070;
//Functional Colors
$color-primary: $color-yellow;
$color-secondary: $color-gray;
$color-text: $color-gray;
//Layout
$max-width: 1200px;
$header-height: 80px;
$header-height-big: 100px;
// Spacing
$gutter-padding: 12px;
// Mobile Breakpoints
$mobile-screen: 160px;
$small-screen: 767px;
$medium-screen: 992px;
Once you are done with above changes deploy the code in AEM and drag and drop the component on SPA page. Below is the final snap of the component that’s created. We can customize the react code to add more responsiveGrid as well and arrange them in Horizontal order.
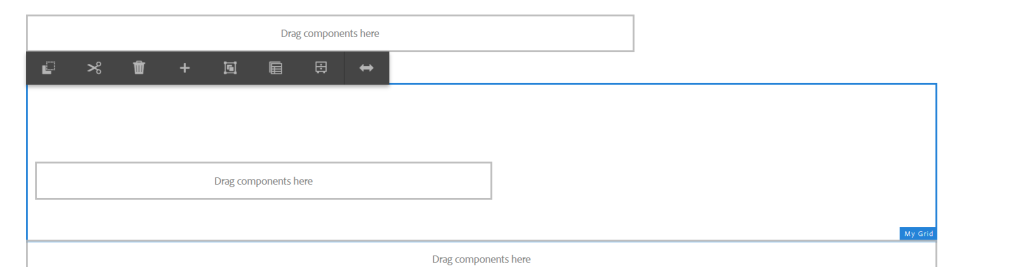
Comment or DM me in case of any clarification or update on the article.